Developing Backbone.js Applications. Building Better JavaScript Applications Addy Osmani

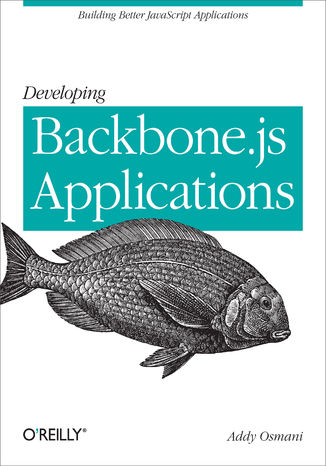
- Autor:
- Addy Osmani
- Wydawnictwo:
- O'Reilly Media
- Ocena:
- Stron:
- 374
- Dostępne formaty:
-
ePubMobi
Opis
książki
:
Developing Backbone.js Applications. Building Better JavaScript Applications
If you want to build your site’s frontend with the single-page application (SPA) model, this hands-on book shows you how to get the job done with Backbone.js. You’ll learn how to create structured JavaScript applications, using Backbone’s own flavor of model-view-controller (MVC) architecture.
Start with the basics of MVC, SPA, and Backbone, then get your hands dirty building sample applications—a simple Todo list app, a RESTful book library app, and a modular app with Backbone and RequireJS. Author Addy Osmani, an engineer for Google’s Chrome team, also demonstrates advanced uses of the framework.
- Learn how Backbone.js brings MVC benefits to the client-side
- Write code that can be easily read, structured, and extended
- Work with the Backbone.Marionette and Thorax extension frameworks
- Solve common problems you’ll encounter when using Backbone.js
- Organize your code into modules with AMD and RequireJS
- Paginate data for your Collections with the Backbone.Paginator plugin
- Bootstrap a new Backbone.js application with boilerplate code
- Use Backbone with jQuery Mobile and resolve routing problems between the two
- Unit-test your Backbone apps with Jasmine, QUnit, and SinonJS
Wybrane bestsellery
O'Reilly Media - inne książki
Dzięki opcji "Druk na żądanie" do sprzedaży wracają tytuły Grupy Helion, które cieszyły sie dużym zainteresowaniem, a których nakład został wyprzedany.
Dla naszych Czytelników wydrukowaliśmy dodatkową pulę egzemplarzy w technice druku cyfrowego.
Co powinieneś wiedzieć o usłudze "Druk na żądanie":
- usługa obejmuje tylko widoczną poniżej listę tytułów, którą na bieżąco aktualizujemy;
- cena książki może być wyższa od początkowej ceny detalicznej, co jest spowodowane kosztami druku cyfrowego (wyższymi niż koszty tradycyjnego druku offsetowego). Obowiązująca cena jest zawsze podawana na stronie WWW książki;
- zawartość książki wraz z dodatkami (płyta CD, DVD) odpowiada jej pierwotnemu wydaniu i jest w pełni komplementarna;
- usługa nie obejmuje książek w kolorze.
Masz pytanie o konkretny tytuł? Napisz do nas: sklep@ebookpoint.pl
Książka drukowana

Oceny i opinie klientów: Developing Backbone.js Applications. Building Better JavaScript Applications Addy Osmani
(0)